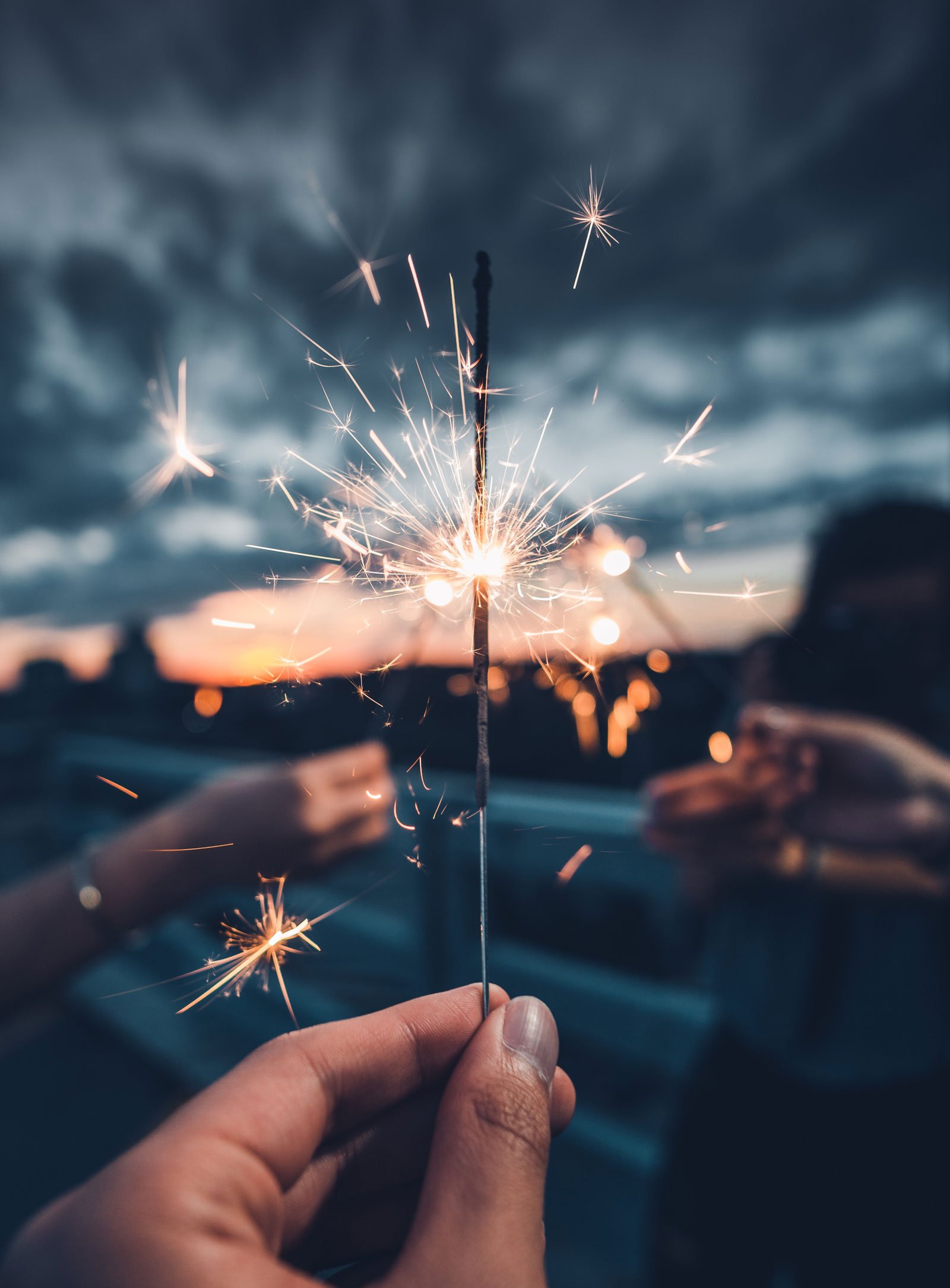
Ever feel like you are repeating tasks all day long? Caching is a powerful technique when building websites and can help you save time and resources.
What is caching?
Caching is simply storing a computed output based on input--giving the
ability to retrieve the stored output without having to go through the
computation again. It can be a powerful technique when building websites and is sometimes overlooked.
Caching is very important when it comes to building websites. Imagine having a math test that asks a math question multiple times (yes, I know that sounds stupid, but just imagine it!). If you’ve already answered the question once (hopefully correctly), then there is no need to do the problem over again when you see it. You can simply copy the answer from the previous question (and no, it’s not cheating if it’s your answer). This is the whole point of caching. When building websites, it is important to use caching, because it frees up resources and time. This is especially true if the website uses a database to get information. Caching the output of a select query, for example, will not only free up your database from having to do the work again, but it will also free up network traffic that is generated by transferring the data over to your web application, thus speeding up the
performance for your users, because they will get the cached results faster.
How to Cache
Let’s say you have a function that multiplies two numbers:
float Multiply(float x, float y)
{
return x * y;
}
This function isn’t using caching, so each time it’s called, it will multiply x and y again, even if it has done so previously.
To implement a caching version, there is a simple pattern that can be applied. Simply check to see if there is a cached version of the input. If there isn’t, do the computation and store it into the cache. If there is a cached version of the input, simply return the cached version. It is important to notice that the cache key is based on the function name and input values. This is a simple technique that can be used to identify and store the cache, because a function usually should return the same output if given the same input.
Below is an example:
float Multiply(float x, float y)
{
string cacheKey = String.Format("Multiply{0}_{1}", x, y);
if (HttpContext.Current.Cache[cacheKey] == null)
{
float z = x * y;
HttpContext.Current.Cache.Insert(
cacheKey, z, null,DateTime.Now.AddMinutes(30),
System.Web.Caching.Cache.NoSlidingExpiration
);
return z;
}
else
{
return (float)HttpContext.Current.Cache[cacheKey];
}
}
When should you cache?
It’s important to know that programming is more of an art than a science. You’ll have to decide whether something should be cached or not. You don’t want to have tons of code that caches everything in your web application, because that will add to needless complexity. Sometimes you have functions that can potentially return different data depending on when they are called, even if the inputs are the same. For example, if you have a database table of all current products, it can change over time. This is when it’s important to look into cache expiration. When building a website, it’s important to take a step back and decide if caching could help the performance of your site. But no matter how extensively you use caching, it’s an essential tool for ensuring that your website runs smoothly and efficiently and provides the best experience for your users. Feel free to contact us at Diagram if you have any questions about how you can improve the performance of your site.
Related Posts
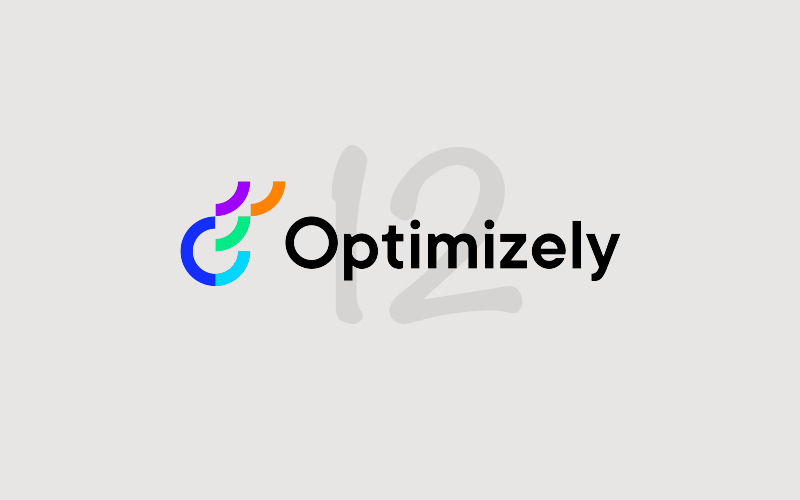
Navigating an Optimizely CMS 12 Upgrade
Learn why an Optimizely CMS 12 upgrade needs detailed planning, efficient resource allocation, and an understanding of your organization's capabilities.
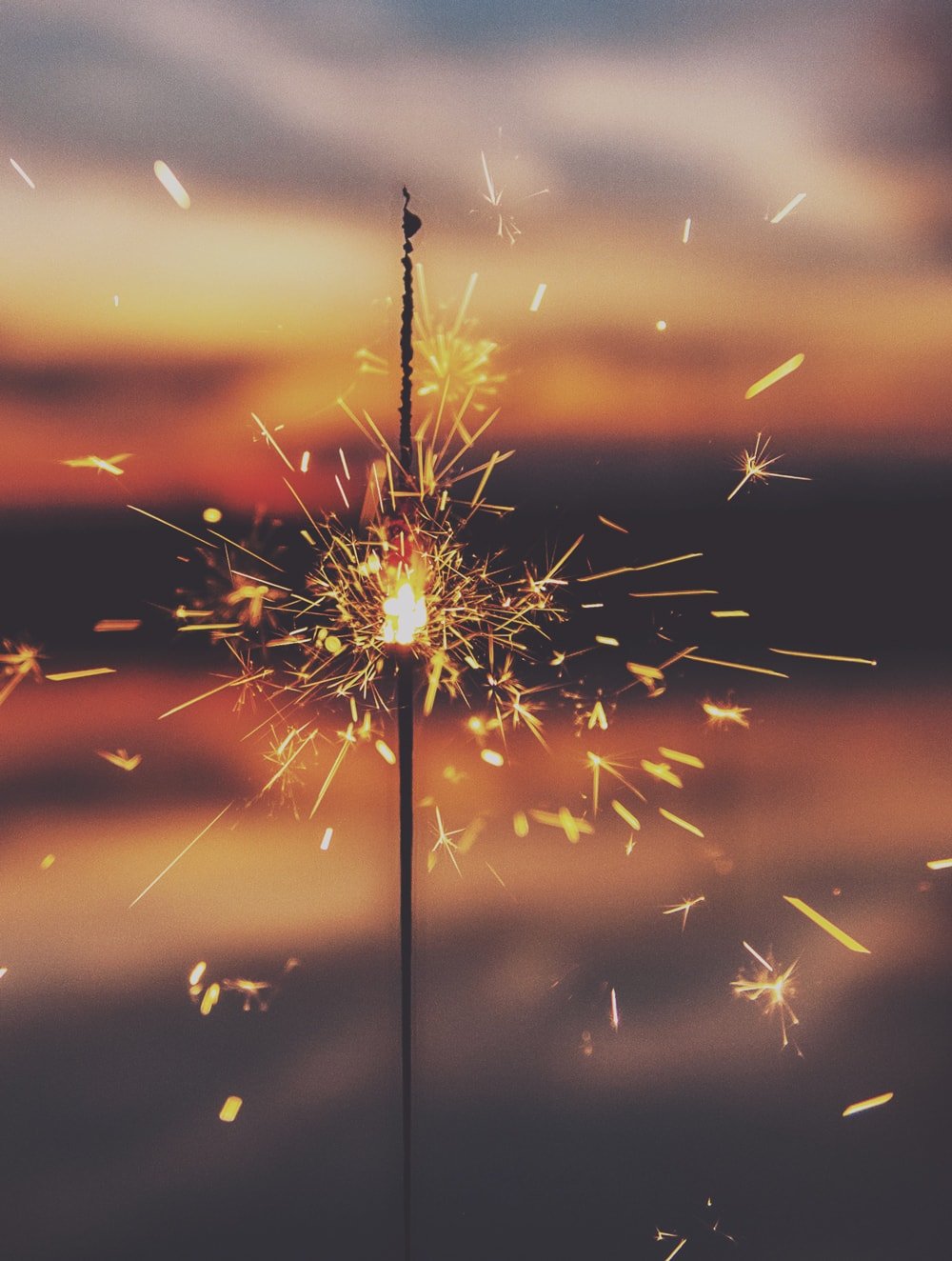
Why Choose a CMS?
We look at the advantages that a Content Management System (CMS) can bring to your digital and content marketing strategy.
Results Matter.
We design creative digital solutions that grow your business, strengthen your brand and engage your audience. Our team blends creativity with insights, analytics and technology to deliver beauty, function, accessibility and most of all, ROI. Do you have a project you want to discuss?
Like what you read?
Subscribe to our blog "Diagram Views" for the latest trends in web design, inbound marketing and mobile strategy.